Unlocking the Power of Web Scraping with PHP and cURL
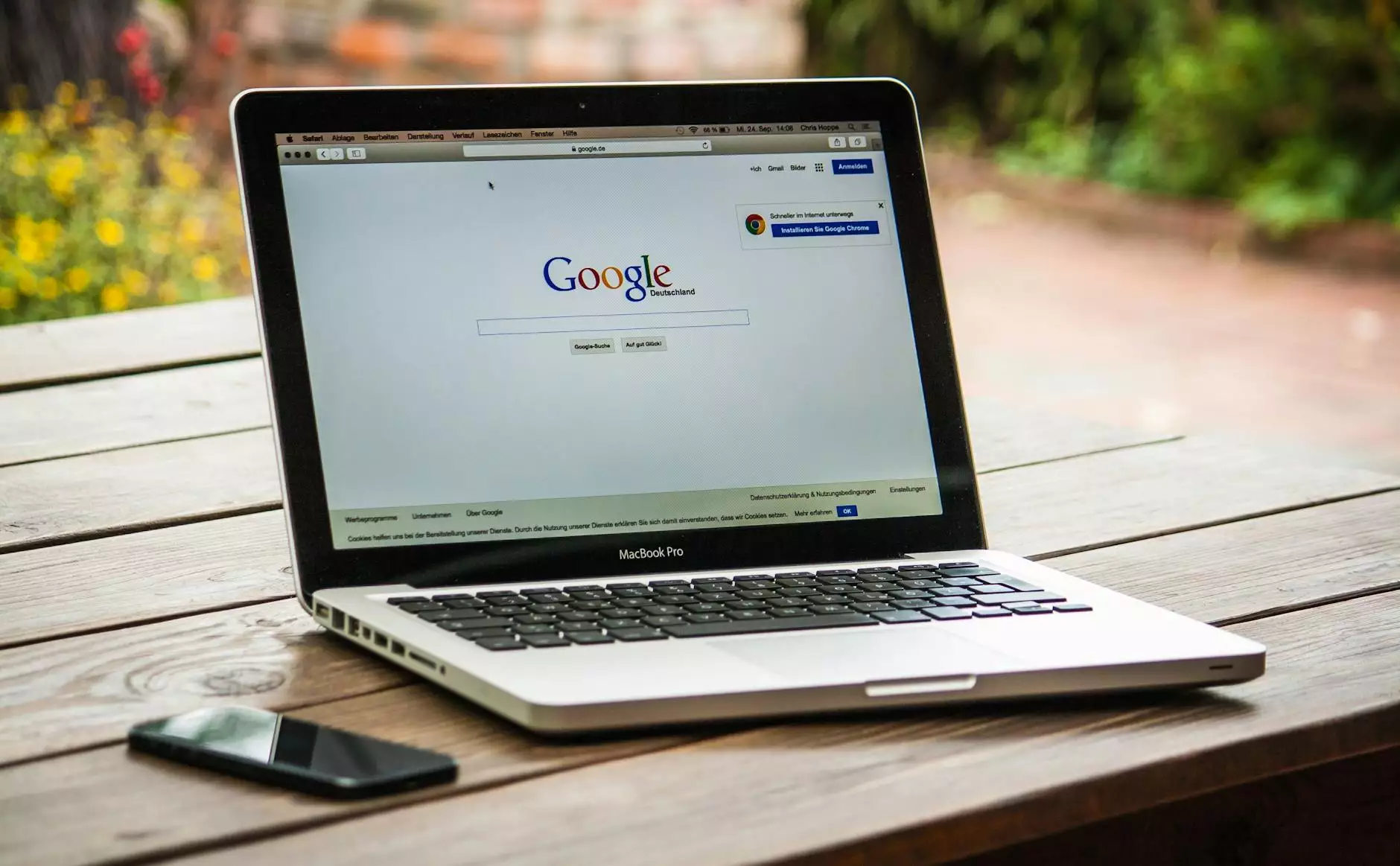
Web scraping is a powerful technique that allows businesses to gather data from various web sources effectively. In this guide, we will explore the process of web scraping with PHP and cURL, providing comprehensive insights and practical examples to help you harness this technology for your business needs.
What is Web Scraping?
Web scraping is the process of automatically retrieving and extracting data from websites. This practice is essential for businesses seeking to collect data for diverse purposes, such as:
- Market Research: Understanding competitors and market trends.
- Price Monitoring: Keeping track of product prices across different websites.
- Lead Generation: Collecting information on potential customers and clients.
- Content Aggregation: Compiling content from various sources into one platform.
With the right tools and techniques, web scraping can be an invaluable asset for digital marketing, e-commerce, and various other fields.
Why Choose PHP and cURL for Web Scraping?
When it comes to web scraping, many programming languages can be utilized. However, PHP paired with cURL stands out for several reasons:
- Ease of Use: PHP’s syntax is user-friendly, making it accessible for beginners and experienced programmers alike.
- Server-Side Language: Being a server-side language, PHP can perform operations effectively without client-side limitations.
- cURL Library: The cURL library enables users to make HTTP requests and handle various protocols with ease.
- Community Support: A large community of PHP developers ensures a wealth of resources, tutorials, and support.
Setting Up Your Environment for Web Scraping with PHP and cURL
Before you can dive into web scraping, it's crucial to set up your development environment. Follow these steps to get started:
- Install PHP: Make sure you have PHP installed on your local machine or server. You can download it from the official PHP website.
- Enable cURL: Ensure that the cURL extension is enabled in your PHP installation. You can check this by running phpinfo(); and looking for a section labeled 'cURL'.
- Choose an IDE: Select an Integrated Development Environment (IDE) or code editor that suits your workflow. Popular choices include Visual Studio Code, PhpStorm, or Sublime Text.
Understanding the Basics of cURL
cURL stands for "Client URL" and is a command-line tool used to send and receive data to and from servers. It supports various protocols, including HTTP, HTTPS, FTP, and more. In PHP, you can use cURL functions to initiate requests, set options, and handle responses.
Basic cURL Functions
Here are some essential cURL functions you should be familiar with:
- curl_init(); - Initializes a new cURL session.
- curl_setopt(); - Sets options for a cURL transfer.
- curl_exec(); - Executes the cURL session and returns the response.
- curl_close(); - Closes the cURL session and frees resources.
Step-by-Step Guide to Web Scraping with PHP and cURL
Now, let's dive into the actual process of scraping a website using PHP and cURL. We’ll go through a simple example to illustrate the concepts better. For this example, we will scrape blog titles from a travel blog.
1. Initializing cURL
Start by initializing cURL in your PHP script:
2. Setting cURL Options
Next, set the necessary options for the cURL transfer:
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true); ?>CURLOPT_RETURNTRANSFER tells cURL to return the response as a string instead of outputting it directly, while CURLOPT_FOLLOWLOCATION allows redirection if the URL responds with a redirect status.
3. Executing the cURL Request
After setting the options, execute the cURL request:
$response = curl_exec($ch); ?>4. Checking for Errors
Always check for errors after executing a cURL request:
if (curl_errno($ch)) { echo 'Error:' . curl_error($ch); } ?>5. Closing the cURL Session
Once you have finished the request, close the cURL session:
curl_close($ch); ?>6. Parsing the Response
The next step involves parsing the HTML response to extract the required data. For this, you can utilize PHP's DOMDocument class or libraries like simple_html_dom.
libxml_use_internal_errors(true); // prevent warnings $dom = new DOMDocument(); $dom->loadHTML($response); $titles = $dom->getElementsByTagName('h2'); // assuming the titles are in tags
foreach ($titles as $title) {
echo $title->nodeValue . "";
}
?>Best Practices for Web Scraping
When scraping data from websites, it’s essential to follow best practices to avoid legal issues and ensure that your scraping is efficient and effective.
- Respect Robots.txt: Always check the robots.txt file of the target website to see if they permit scraping. This file specifies which parts of the site can be crawled by automated agents.
- Implement Throttling: Avoid overwhelming a server by adding delays between requests (e.g., using sleep(1); in your script).
- Keep User-Agent Random: Alter your User-Agent string to mimic different browsers and avoid detection.
- Data Storage: Choose a suitable method to store your extracted data, such as databases, CSV files, or JSON files.
Conclusion
Web scraping is a valuable technique for collecting data that can fuel business growth and strategic decision-making. By mastering web scraping with PHP and cURL, you can unlock a world of data at your fingertips.
As you implement web scraping in your projects, remember to adhere to ethical practices and legal guidelines. Dive deep into the possibilities, and embrace the insights that data can provide for your business journey.
Further Resources
Continuously expand your knowledge about web scraping and programming by exploring these resources:
- PHP cURL Documentation
- W3Schools cURL Tutorial
- Tutorials Point on PHP Web Scraping